Strange Debugger Issue with Local Variables
Update: tested against the latest XCode 4.2 build and it is still the case. So I reported a bug:
Update: after I wrote and published this entry I realized that what I suspected might be right. So I made a breakpoint inside the constructor of the class in question: ProgramStepEntity and later when it is used as local variable. Now all the variable show up in the constructor (actual init method) as well as when it is used as local variable. Now I don’t want to have breakpoints in every constructor just to make sure that the debugger is working correctly. Need to check if that is still the same problem in 4.2 and if yes then I’ll fill a bug report.
Since a while I am bogged with a stupid debugger issue using XCode 4.0.2. So what gives:
In XCode4 you don’t have to declare your variables anymore if you declare them as properties (@property …). The compiler will take the info from the property definition and declare the variable. That works fine and the properties will show in the debugger when they are inside self or a member variable of self. So far so good.
But when I use the same class as a local variable the debugger is not showing that member. This is the code:
@interface ProgramStepEntity : BaseKVCMapper {
}
@property (nonatomic, retain) NSString* instruction;
@property (nonatomic, retain) NSArray* parameters;
@property (nonatomic, retain) NSString* target;
Here is another break point where the variable show up:
This can be fixed if I declare the variable:
@interface ProgramStepEntity : BaseKVCMapper {
NSString* instruction;
}
@property (nonatomic, retain) NSString* instruction;
@property (nonatomic, retain) NSArray* parameters;
@property (nonatomic, retain) NSString* target;
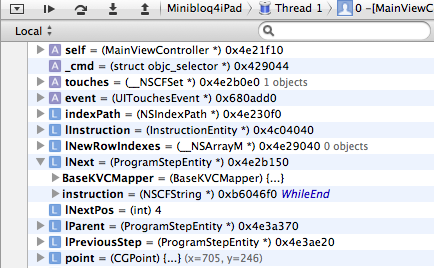
Now sure what is wrong but I sometimes see all the variable will show up and sometimes they don’t. It could be that the debugger is storing the list of variable once and if at that time the list is incomplete it will not show the rest.
– Andy
XCode4: a Big Step in iOS Development
Yesterday Apple released XCode4, the IDE (integrated development environment) to develop iOS and Mac OS X Applications. After more than 1 year (as far as I can remember) this finally will make developing iOS apps much easier and I think much faster and definitively more fun.
Beforehand in XCode3 the Interface Builder was separated from XCode and created many windows which were hard to track and especially harder to close. Just opening a few XIBs and the screen was cluttered with windows all over the place. The only way to managed them was to close the IB from time to time. Now in XCode4 the IB is integrated into XCode and is part of its UI. Due to the fact that XCode is now a single window application there are no more floating windows around. The only other windows one might see are other applications or the Organizer window which contains the help pages, source control systems, devices, projects and archives.
But that is not everything. Because IB is part of it one can have the UI on the left hand editor and display the related View Controller header class file on the right hand editor. This way it is easy to add new IBOutlet properties and IBAction methods without having to leave the UI editor. This works the same way as when I open the implementation class (.m) and have set the right editor to be on counterpart then it will display the header class (.h) or vice versa. The right window can also be used to display the differences in the versions of one file.
The right sidebar is used to display the File Inspector and Quick Help and if an XIB is opened it displays the Identity, Attribute, Size and Connection Inspectors as well.
Beside that the Editor itself has improved dramatically with better code completion, error highlighting as well as code snippets. This improves the overall quality of the code written.
The bottom bar is used to display the log files when the app is running as well as the debugger controls. This way I don’t have to search for the Output and it can be easily viewed while the app is running.
Having used IntelliJ IDEA for a long time the XCode is coming close to it making developing Apps much easier and prevents me from juggling a million windows. I have used XCode4 since more than half a year and so I am very happy to see it get publicly released.
That said there is a ton of more functionality that I did not encountered yet or have not used it so I am looking forward to use it even more and share my thoughts with others.
-Andy
Animate a Blocking Dialog in iOS
Today I was faced with a little challenge when I discovered that a blocking Help Dialog which should slide on and off does not animate after the user clicks OK.
It turns out that the UIView animation is executed in a background thread and so the method that is executed when the user clicks OK is processing further without waiting for the animation to finish. Within there I will yank the view from its parent and so the animation is not shown.
The solution to this is to register an Animation Delegate as well as the method that is called when the animation is done. This is done this way:
[UIView beginAnimations:@"hideView" context:NULL];
...
[UIView setAnimationDelegate:self];
[UIView setAnimationDidStopSelector:@selector
(animationDidStop:finished:context:)];
ATTENTION: Please note that these settings must be done within the animation block. Setting them outside means nothing is going to be happening.
This informs the given delegate when the animation is done and there is where I can release the block:
- (void)animationDidStop:(NSString*)animationID
finished:(BOOL)finished
context:(void *)context
{
CFRunLoopStop( CFRunLoopGetCurrent() );
}
This will do the trick and allow me to clean up the dialog meaning removing it from the super view so that it will eventually released and not become a memory leak.
This little challenge also means that I finally become some sort of a iOS crack delving deeper and deeper into regions of iOS is never dared before to even have a look at it.
Cheers – Andy
iPhone Crashes in the Nowhere Land
On Sunday I started to have a closer look at the memory management of the application and fully implemented the dealloc methods. Subsequent testing revealed a nasty bug where the application just died when I moved from one part of the application do another but I had not idea why it was crashing and especially where.
Well, at least I had a hunch where it was happening XCode did not point near there. Eventually I started to set breakpoints in all the dealloc methods that I could think of would be called. Eventually I saw that one property was already released and my release made it crash. So I just tried a few things and eventually found the resolution in calling the super class’ dealloc method at the end. So far I did not find any clear instruction if to call it first or last but it seems to be that way that it needs to be called last.
There are a few other things I have to keep in mind when dealing with memory management:
- Use properties when dealing with references because it does handle re-assignments by itself
- With properties every assignments to a property must be done with self like this: self.myProperty = …. This way we make sure that the setter method is called and subsequently the object is retained (if marked as such)
- Called [super dealloc] as last statement in the object’s dealloc method (if provided)
- Every object creation with alloc or new must be autoreleased when assigned to retaining property
Well, no I can go back and chase some more bugs.
-Andy